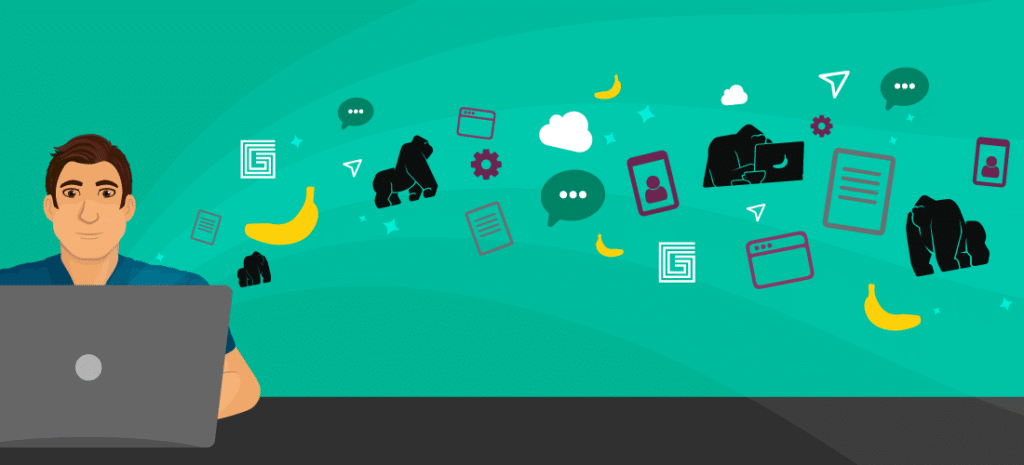
How to Build a Real-world App: A Nuxt.js Tutorial (Part 1)
Nuxt.js is called the intuitive Vue.js framework. It’s open source, simple, and quite powerful, with more than 30 thousand Github stars and about 50 modules already built so you don’t have to reinvent the wheel. But wait, there’s more! It uses all the best practices from Vue.js and Node.js, and creates an excellent developer experience. I think you’ll find that the real value is in all the time you can save during front-end implementation. The documentation is excellent, making it easy to understand and maintain a modular implementation. Interested in learning about Nuxt.js? In this Nuxt.js tutorial, we’ll provide a very basic introduction to what it can do, through a project that will consume a blog post API endpoint and then display it.
Step 1: Create the project with CLI
To get started, you’ll need Node.js version 10 or later installed in your machine. Also using the Vue.js devtools for Chrome will help, so create a directory where the project is going to live and then using the CLI, add:
$sudo npm i -g create-nuxt-app@2.1.1
(to avoid any conflict use 2.1.1 for now)
$create-nuxt-app gorilla-news
(you can choose the project name you want)
For options:
• no server and UI framework are required
• rendering mode is Single Page App, yes for axios and prettier, no for eslint, and npm for package manager
From inside your project directory, run:
$npm run dev
You will see an initial page with the Nuxt.js logo.
Step 2: Add Vue-Material
Vue Material helps us to make our app look good and save time when we add styles, so let’s install it:
$npm i vue-material
Next, go to the plugins folder, create a new file vue-material.js, and add the following lines to it:
import Vue from 'vue'; import VueMaterial from 'vue-material'; Vue.use(VueMaterial);//to register globally
We need to let Nuxt.js know that about this plugin, so go to the nuxt.config.js file and add “vue-material” inside the plugins array, like this:
{ src: '~/plugins/vue-material'}
We also need to add this inside the css array:
{ src: 'vue-material/dist/vue-material.min.css', lang:'css' }
For our project example, we’ll use icons, so we’ll add the Material Icons library (same nuxt.config.js file) inside the link array, in the head section (remember to add commas to separate array elements):
{ rel: 'stylesheet', href: '//fonts.googleapis.com/css?family=Roboto:400,500,700,400italic|Material+Icons'}
Now, what do we need to generate a theme? Sass should be a good start, so let’s install it:
$npm i -D node-sass sass-loader
Next, we need to create a file call: theme.scss inside the assets directory. This will import the material engine, and also include the register for our new theme.
There are some configuration options for the theme, and you can choose the ones that you like. Go to Vue Material–Themes-Configuration and copy the first piece of code inside the theme.scss file. Remember to add that Sass file as a new CSS reference in the css array ,like this:
{ src: '~/assets/theme.scss', lang:'scss' }
Step 3: Consume the API
Now we’re ready to consume any blog post API to display it. In this example, we’ll consume the official blog post feed from Gorilla Logic: /wp-json/wp/v2/posts?_embed (but of course you can use any API you want, the only requirement for our purposes is that it contain images URL in it). Another option you have is the API key/credentials. In our example, we don’t need it. To consume the API, we are going to use one of the most common plugins in Vue.js: Axios. Please create an axios.js file inside the plugins folder. This is an important step of this Nuxt.js tutorial!
In order to consume the blog post from the API, you must use the asyncData function, return an array with the data, and display each element using a v-for loop. The code looks something like:
(Reference: https://github.com/kgatjens/gorilla-nuxt-blog/blob/master/pages/index.vue)
Now in the CLI run:
$npm run dev
(in case it wasn’t up)
And the returned data might look like:
That’s the basic idea: consume and display data using axios inside the main index.vue page.
Let’s add the proxy module, because it will help us to manage the API endpoints in a more elegant way. For example, instead of always copying the entire URL, we would just add “/api/” .
In the CLI, run:
$npm i @nuxtjs/proxy
Add a reference from this newly installed module in the main config file (nuxt.config.js), inside the modules array.
Also add a “proxy:true, credentials: false” inside the axios config array.
Next, create a new separate “proxy” config array. It should look something like this:
The idea is to manage the “Base API URL” as a target; then, at the time that we call “api” in the pages, Nuxt will know that this is a direct reference to our main API endpoint.
Step 4: Adding layout
You have made it to the final step of this Nuxt.js tutorial! Inside the main index.vue page, we need to add a container for every post. To do that, we can use the material layouts styles. Keep the original v-for statement and add “md-” styles (check Vue material) so that we can make sure that the app displays well on mobile.
For our example:
• Each element must display image, title, and some icons
• Everything should be inside the md-card tag, and inside of that, the tags: md-card-media, md-card-header, md-card-content will create the cards for each blog post
Basic HTML is added internally with the title, author name, and a few icons.
Important: Keep in mind that you should use the new “post” object correctly, calling the data fields such as title, author, and image source property. You can add id if your API endpoint has it. The card code looks something like this:
To recap, we now have an index page where we can see the list of blog posts. We’re getting the information from the API using only the basic setup of axios.
After adding some material tags and classes, we should be able to see the cards like this:
Wrapping up the first part of our Nuxt.js tutorial
And there you have it–we’ve learned from our simple Nuxt.js tutorial how to:
• Create a basic setup from Nuxt.js using CLI
• Create a theme
• Configure a layout setup using Vue-Material
• Understand how Nuxt.js manages routes
• Manage an API endpoint call using Axios
If you spend a few hours exploring, you’ll quickly get an idea of how this framework works and how it can help in your upcoming projects.
In the second part of this Nuxt.js tutorial, we’ll create basic register functionality and connect it to Firebase, including how to:
• Create a main nav for our app
• Create a new “register” page
• Use the email/password API functionality from Firebase and connect our register with it
• Display an avatar as soon as any user is registered by checking the store values