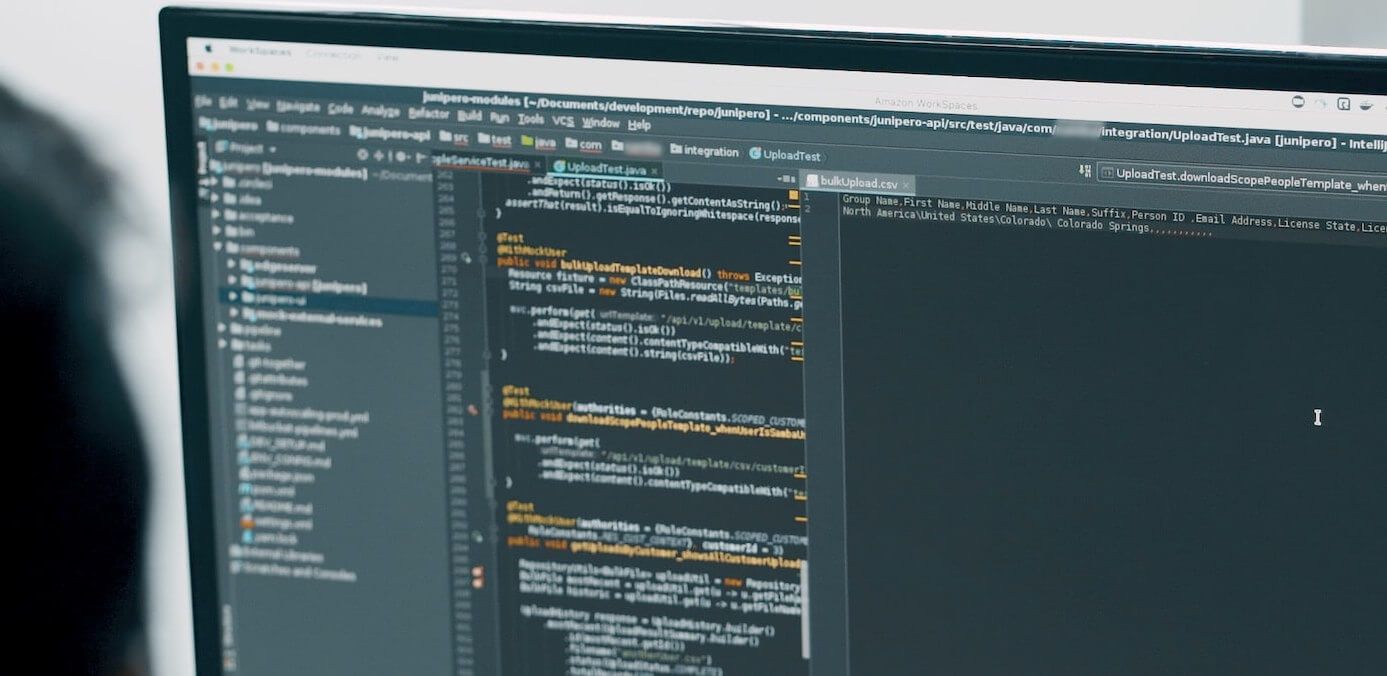
Creating a Simple Website: A Gatsby Tutorial With WordPress and GraphQl
In this Gatsby tutorial, we will create a simple news website, MTB News, using Gatsby.js to render static pages on the server-side consuming a WordPress API with basic content via GraphQL. Through this blog, you will:
• Understand Gatsby
• Install Gatsby helpers using the command line interface
• Use GraphQL, a query language for APIs that Gatsby uses, to perform simple queries
• Create components to experiment with in React
• Use WordPress as an API
• Create new content types in WordPress
Let’s get started!
What is Gatsby.js?
Gatsby.js is a progressive web app (PWA) generator. It’s got a well-deserved reputation for being very fast. It’s fast because it loads only the critical HTML, CSS, data, and JavaScript for your site. Gatsby generates static page files using React as the main engine. Using Gatsby, you can:
• Build really fast websites, with pages delivered instantly.
• Build websites by consuming data from many different sources including CMSs, SasS services, APIs, databases, and more.
• Deploy static generated pages easily on many cloud-hosting services.
• Access ReactJS and Webpack already all set up and ready to use.
• Get all the advantages of JAMstack (JavaScript + APIs + Markup), including better performance, higher security, and an easy developer experience.
If you need high performance and your content will not change often, consider Gatsby a must-have.
Installing WordPress
For our simple Gatsby tutorial, you’ll need a place to manage your content. We’ll use WordPress (WP), so you need the following:
• WordPress installed on a local machine
• An Apache or Nginx server
• A MySQL or MariaDB server
For help configuring this basic setup, check out this manual.
Note that for this example:
• WordPress will run in http://localhost:8888/
• From the CMS or API, the URL will be http://localhost:8888/gatsby/demo1/wp-json/wp/v2/
.
Installing Gatsby
1. Install the Gatsby server:
$ npm install -g gatsby-cli
$ gatsby new mtb_website
: Create the Gatsby site.
$ gatsby develop
: Start the development server.
2. Run your Gatsby setup at: http://localhost:8000/
For more information see: https://www.gatsbyjs.org/docs/quick-start/
Important Installs in WP to Start Using GraphQL
Now let’s explore GraphQL.
1. Go to: https://www.gatsbyjs.org/plugins/ and search for: gatsby-source-wordpress
2. Stop the Gatsby server in your CLI (Ctrl + c) and install: $ npm install --save gatsby-source-wordpress
3. Go to your new Gatsby directory project and inside the gatsby-config.js
include the reference for the last added plugin: gatsby-source-wordpress
Go to: https://www.gatsbyjs.org/packages/gatsby-source-wordpress/#how-to-use
Copy ALL of the code below as a new plugin in that file without overwriting anything.
4. Test that WP is responding with an API. You can use URLs like: http://localhost:8888/gatsby/demo1/wp-json/wp/v2/
5. If WP is responding, change the baseURL for your previous local WP installed inside gatsby-config.js .
In my example, I’ll add something like: http://localhost:8888/gatsby/demo1
6. Run $gatsby develop
in your terminal inside your Gatsby folder project path.
7. Under http://localhost:8000/
look for the default Gatsby Starter interface.
8. You can display the GraphQL explorer with: http://localhost:8000/___graphql
9. Try a basic query like this:
Create Pages and a Menu
Now we let the system create pages dynamically:
1. Create a directory with the name: templates inside the src main folder.
2. Create two files: post.js and page.js
3. Install $ npm install --save bluebird
This library helps manage the callback or Promise from pages. We’ll generate templates for pages and posts using React. Then, Gatsby-node will take the data and send it to the specific template for rendering the page content.
For more information about how to manage the gatsby-node.js file to create pages programmatically, see this document.
You can copy the code for this example from here.
4. To retrieve the menu as an API so that it can be used and displayed inside the Gatsby section, install:
https://wordpress.org/plugins/wp-api-menus/ in your WP installation.
5. Activate it and then you can access the menus items as an API like this:
http://localhost:8888/gatsby/demo1/wp-json/wp-api-menus/v2/menus/
Remember that to create a menu first, you just need to go inside the WP setup and create a really basic menu via Appearance>Menus. For this example, we created “Main Menu,” the Home page, and a previously created “News” page.
6. Let Gatsby know that it should consume the new menu. Go to Gatsby-config.js, and in includedRoutes: [ ] add a route for the menus (“**/menus”,) and restart the server.
7. GraphQL should now have access to the menus in the MyQuery:
allWordpressWpApiMenusMenusItems
8. Create the Menu components inside src/components/. Create a new file MainMenu.js. This is where the main menu layout will be.
The StaticQuery object from Gatsby will let you get the menu items, manage them using a loop, and display them.
The Link object and the GraphQL are also required: import {graphql, StaticQuery, Link} from 'gatsby';
Styling the Main Menu
To save time, we’ll use Styled Components for our basic menu.
1. Install Styled Components with npm using your CLI:
$ npm install --save gatsby-plugin-styled-components styled-components babel-plugin-styled-components
Here’s a good guide to Styled-Components : https://www.gatsbyjs.org/docs/styled-components/
2. Add this import in the MainMenu component:
import {createGlobalStyle} from 'styled-components';
A nice trick is to create a const component that includes the styles instead of using the reserve Link component. The most important thing is to get the data (links items) with a query and render all of them:
3. Check for the entire file here.
4. To start using our layout instead of default Gatsby we must clear the layout.js file inside components. Components like <
footer> and <Header>
can be deleted. The MainMenu should be imported, and the global styles can be added. Check for the file here.
5. To complete and display a decent header, we must show the information that’s important for the user, such as the title. The simplest way to do this is to get the title data from the CMS and create a specific component for it.
Take a look in the BasicInfo.js component. It includes the GraphQL query that retrieves the information and fills in the html that will be included in the MainMenu.js header.
Create the MTB News Content Type and a Component for It
The next step within this Gatsby tutorial is to create and render the MTB News content type:
1. Inside the CMS, go to: Appearance > Theme Editor > Theme Functions(functions.php), and add this code:
add_theme_support( 'custom-logo' ); add_theme_support( 'menus' ); add_theme_support( 'post-thumbnails' ); function create_custom_mtb_news_post_type(){ $args = array( 'labels' => array('name'=>__('MTB News'),'singular_name'=>__('MTB News')), 'public' => true, 'show_in_admin_bar' => true, 'show_in_rest' => true ); register_post_type('mtb_news',$args); add_post_type_support('mtb_news', array('thumbnail','excerpt')); } add_action('init', 'create_custom_mtb_news_post_type');
2. Back in WordPress, you should see a new option: MTB News.
3. Click on MTB News, and add a few news items and images in the Featured Image field.
4. The new endpoint is: http://localhost:8888/gatsby/demo1/wp-json/wp/v2/mtb_news
and you need to add it as an includedRoutes in the gatsby-config.js (“**/mtb_news”).
5. Create a component to render the new items (the MTB News items). We need the query to get that information, and then we need to create a new component that we can call: “ MtbItems.js ” inside the components directory. That new component will need a query to get the new MTB content type, like this:
6. Inside that component, we need a very basic layout with a loop for rendering the content and the featured image. Follow this guide as a code example.
7. Add a template that Gatsby can use to display our new content type. If you go to gatsby-node.js, you will notice that Gatsby creates templates for Pages and Posts. We can use similar code to create a template for our new Item:
a) Go to src/templates/ and create a new file call: mtb_news.js. You can just copy and paste from the page.js file, import from styled-components (import styled from ‘styled-components’;) and create a component to style and display the FeaturedImage.
For more information, go here.
b) Inside Gatsby-node.js, completely copy an object similar to PAGE. That section should include the GraphQL query that manages: allWordpressWpMtbNews, updates the page template to: const newsTemplate = path.resolve("./src/templates/mtb_news.js")
and the path to: path: `/mtb_news/${edge.node.slug}/` .
For more help on this, go here.
c) Restart the Gatsby server.
d) Check that you can access the MTB news by URL. This will be something like: http://localhost:8000/mtb_news/transcr-2020/
Create the WP Template to Render the MTB News Items
For the last part of our Gatsby tutorial, we’ll create a specific template inside WP, create a relationship between the template and the MTB News content type, and let Gatsby know which front-end template should be used to load and display the data, depending from the previous related theme template.
1. In WP using the WP theme that is Active ( in this example, Twentynineteen) , go to: wp-content/themes/twentynineteen/
2. Create a new PHP file mtb_content.php and add just this code inside: <?php /*Template name: MTB News Item below content */ ?>
3. In WP, go to Pages, select any existing page (in this example, the page is “ News”) that will display the list of MTB Items and change the template for the last one created (MTB News Item), in the Page Attributes.
4. Inside the gatsby-node get and identify the new template that is coming here: edge.node.template in the GraphQL .
If that template is equal to the previously created template ( mtb-content.php ), we must resolve that Gatsby will use the appropriate template for it: src/templates/mtb_news.js.
Update gatsby-node.js inside the ==== PAGES (WORDPRESS NATIVE) ====
as follows:
a) const mtbContentTemplate = path.resolve("./src/templates/mtb_news.js")
b) Update the component
definition to:
component: slash(edge.node.template==='mtb_content.php' ? mtbContentTemplate : pageTemplate),
5. Restart the Gatsby server.
$ gatsby develop
6. Check that all the functionality is working:
a) A home page
b) Main navigation menu with a link to News
c) A News page displaying all the available MTB news items, with a Read More option that opens the MTB News pages.
That’s the very basic website that we’ve built in our Gatsby tutorial .
You can check my repo for the Gatsby part here.
Ready to Build Your Own Website With Gatsby?
If you want to build high-performance websites, this Gatsby tutorial provides a simple, yet powerful approach. You’ll find learning Gatsby easy, especially with the very good official documentation. I encourage you to explore the power of Gatsby!
References
https://www.gatsbyjs.org/docs/gatsby-cli/
https://reactgraphql.academy/