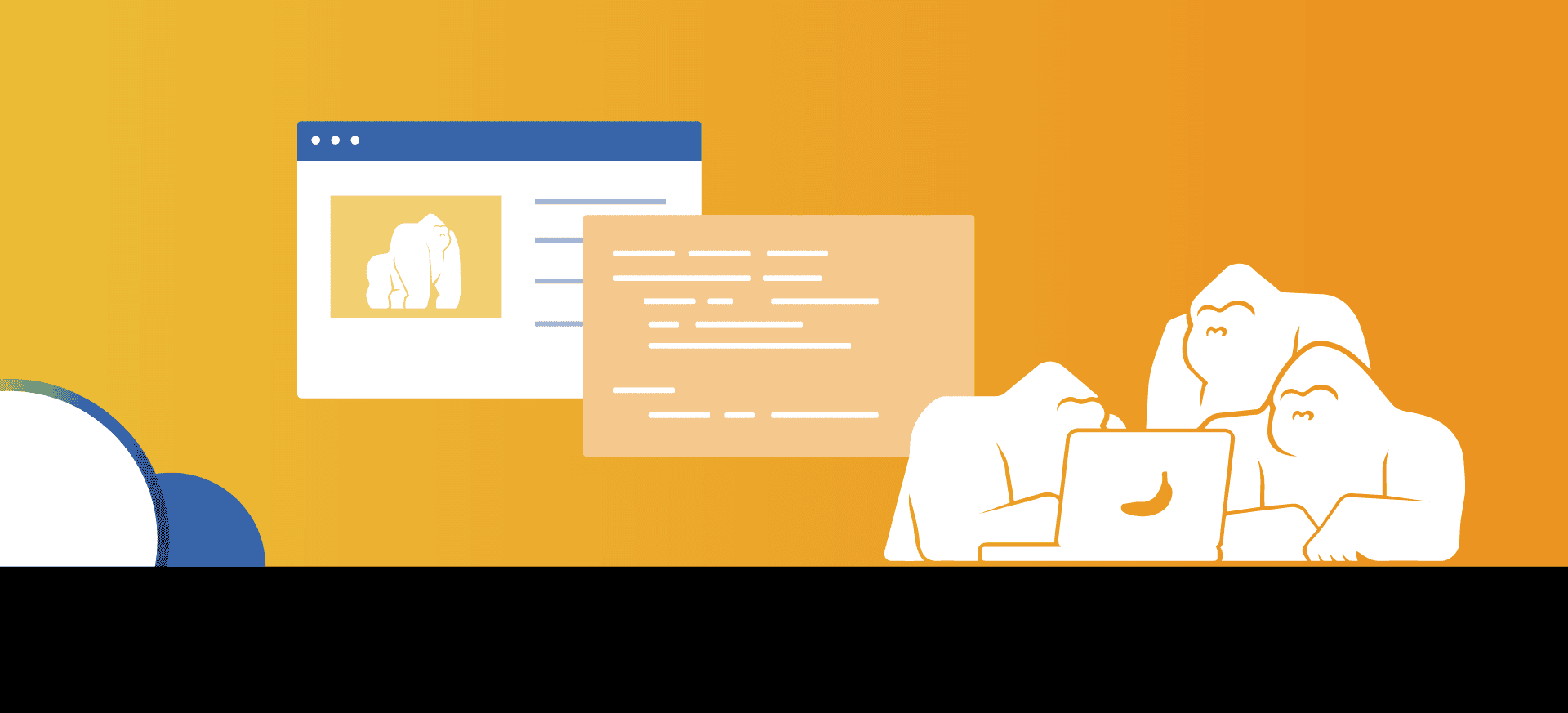
Building Vue Applications with Nuxt.js Part 2: Firebase
This is the second part of a three-part blog series that will introduce you to Nuxt.js, a higher-level framework that builds on top of Vue. In Part 1 of this tutorial, we built a basic structure for our project. In this second entry, we’ll be adding a back-end in Nuxt.js firebase from which we’ll be pulling our blog data. We’ll also create a form to add and edit blogs and link it with Firebase.
Step 1: Setting up our Create/Edit Form
Building a dialog form
Vuetify provides options to handle forms and dialogs, providing the UI components and exposing the relevant props to control the behavior. Vuetify suggests extending the functionality with libraries like vee-validate or Vuelidate. For this example, we will create a simple dialog with input components to create new Gorilla entries.
<v-row>
<v-col
class="text-right">
<v-dialog
v-model="dialog"
width="500"
>
<template v-slot:activator="{ on, attrs }">
<v-btn
color="primary"
v-bind="attrs"
v-on="on"
>
Add Gorilla
</v-btn>
</template>
<v-card>
<v-card-title class="text-h5 grey lighten-2">
Add New Gorilla Type
</v-card-title>
<v-container>
<v-row
class='px-4'>
<v-col
cols="12"
>
<v-text-field
v-model="gorillaForm.name"
label="Name"
></v-text-field>
<v-textarea
v-model="gorillaForm.description"
label="Description"
rows="2"
></v-textarea>
<v-text-field
v-model="gorillaForm.imageUrl"
label="Image URL"
></v-text-field>
</v-col>
</v-row>
</v-container>
<v-divider></v-divider>
<v-card-actions>
<v-spacer></v-spacer>
<v-btn
color="primary"
text
@click="addGorilla"
type="submit"
>
Add
</v-btn>
</v-card-actions>
</v-card>
</v-dialog>
</v-col>
</v-row>
<script>
export default {
data(){
gorillaForm: {
name: '',
description: '',
imageUrl: ''
},
dialog: false
},
methods: {
addGorilla(): {
// TODO implement logic to add Gorilla
}
}
}
</script>
Let’s take a look at each part.
v-dialog
The v-dialog component takes a model that determines when it is being displayed. We have bound this to a state variable called dialog.
v-slot:activator
The activator slot is provided by the v-dialog component, and it allows us to customize the element that triggers our dialog. For this example, we’ve opted for a button that, when clicked, opens the form dialog.
v-text-field
The v-text-field provides a material field that we can bind to a variable in our state. For this example, we’ve created a simple object that contains all the relevant information for the form.
Step 2: Fetching the data using Firebase
Creating a Firebase Firestore database
To host and persist our data, we will use a simple Firestore database:
1. Go to firebase.google.com and create a new project.
2. Once on the project main screen, click on add Firebase to your web app.
3. Register your app name and store the credentials.
4. Under the Firestore database, create a database.
5. For demo purposes, create the database in test mode (this can be updated later following the instructions here).
6. Create a new collection and create a test document with a name, description, and an imageUrl.
And that’s it! We now have a persisted collection of documents that we can retrieve using the Firebase package.
Installing Firebase with Nuxt
To add Firebase to the Nuxt application, follow the next steps:
1. run npm install: npm install firebase
2. run npm install:npm install @nuxtjs/firebase
3. Add the Firebase configuration under our nuxt.config.js file:
buildModules: [
[
'@nuxtjs/firebase',
{
config: apiKey: '<apiKey>',
authDomain: '<authDomain>',
projectId: '<projectId>',
storageBucket: '<storageBucket>',
messagingSenderId: '<messagingSenderId>',
appId: '<appId>'
},
services: {
firestore: true // Just as an example. Can be any other service.
}
}
]
By configuring this, we will now have an instantiated $fire variable provided by Nuxt with the initialized services specified. More information regarding customization and extension of Firebase with Nuxt can be found here.
Retrieving data
Now that we have a working Nuxt.js Firebase connection, we can create a query to retrieve our data and populate our table from our reducer.
export const state = () => ({
gorillas:[]
})
export const mutations = {
setGorillas(state, gorillas){
state.gorillas = gorillas;
}
}
export const actions = {
loadGorillas({commit}, gorillas){
try{
const docsRef = await this.$fire.firestore.collection('gorillas').get();
const gorillaList = docsRef.docs.map(doc => ({... doc.data(), id: doc.id}));
commit("setGorillas", gorillaList);
}
catch (e) {
return Promise.reject(e)
}
}
}
export const getters = {
gorillas: state => state.gorillas,
}
Then, on our index.vue file, we need to specify that we’re retrieving the Gorilla items array as a computed property:
<script>
export default {
...
computed:{
gorillas(){
return this.$store.getters.gorillas;
}
}
...
}
</script>
When we refresh the page, we’ll see the data retrieved from our database.
Step 3: Creating and Deleting data from Nuxt.js Firebase
Delete document in firebase
Supporting deletion is incredibly easy, thanks to Firebase and our previously created delete action button.
Call the delete method from the data table:
<v-data-table
:headers="headers"
:options.sync="paginationOptions"
:server-items-length="serverSidePlaceholer"
:footer-props="footerProps"
:items="gorillas"
:items-per-page="5"
:loading="loading"
class="elevation-1"
>
<template v-slot:[`item.actions`]="{ item }">
<v-icon
small
@click="removeGorilla(item)"
>
mdi-delete
</v-icon>
</template>
Call the store action from the Vue methods:
...
methods: {
async removeGorilla(gorilla){
await this.$store.dispatch('removeGorilla', gorilla.id);
this.resetPagination();
},
...
In the store:
async removeGorilla(_conf, gorillaId){
try {
await this.$fire.firestore.collection("gorillas").doc(gorillaId).delete();
} catch (e) {
return Promise.reject(e)
}
}
Create a document in Nuxt.js Firebase
Creating a new document is also easy now that we have created a form with all the required information.
First, call the create method from the form:
<v-card-actions>
<v-spacer></v-spacer>
<v-btn
color="primary"
text
@click="addGorilla"
type="submit"
>
Add
</v-btn>
</v-card-actions>
Call the store action from the Vue methods:
...
methods: {
async addGorilla() {
this.dialog = false;
await this.$store.dispatch('addGorilla', this.gorillaForm);
this.resetPagination();
this.gorillaForm = {
name: '',
description: '',
imageUrl: ''
};
},
...
In the store:
async addGorilla(_conf, gorilla){
try {
await this.$fire.firestore.collection("gorillas").add(gorilla);
} catch (e) {
return Promise.reject(e)
}
},
And now we have the ability to create and delete Gorillas:
Wrapping up our simple Nuxt.js firebase tutorial
We’re done! Remember that you can download and check the final repo here.
I hope that you enjoyed this Nuxt.js, Firebase, and Vuetify tutorial. Vuetify adds great usability to an already powerful framework, making it possible to create complex solutions with minimal effort. The customizability and wide array of components make it a perfect companion for projects of any size and difficulty.