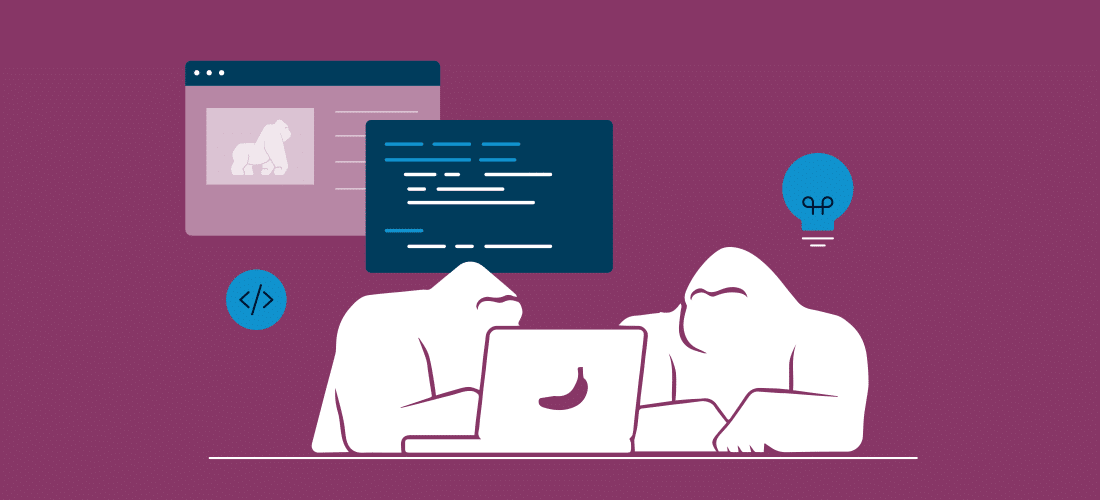
An Easy Way to Automate Web App Testing: Selenium WebDriver Tutorial
Most of us find testing a web application to be very time-consuming and complex because there are so many scenarios to consider. If you automate testing with scripts, you can do the work once and then execute the script whenever you need to test, with little or no manual intervention. This approach can save a lot of time. It can also help improve your testing process.
One of the best and most popular tools for automating web application testing is Selenium WebDriver, a part of an open-source suite of tools. You can use Selenium WebDriver to automate and execute tests against different browsers and platforms. This blog provides a Selenium WebDriver tutorial, which will help you configure the tool for the first time and learn some basic scripting you can use with the tool.
Installing and Configuring Selenium WebDriver
1. First, install Java, if it’s not already present:
• Download and install the Java Software Development Kit (JDK) here.
• Choose the JDK best suited for your system:
• Accept the License Agreement and download the software.
• Open a command line prompt and type “java -version”.
You should see something like this:
2. Install the Eclipse IDE:
• Download Eclipse IDE for Java Developers here.
• Go to the Downloads folder and start the executable file – eclipse-inst-win64, then select Eclipse IDE for Java Developers:
• Install and Launch the Eclipse IDE
3. Install the Selenium driver files:
• Download the Selenium Java Client Driver here. You will find client drivers for many languages there. Choose the one with Java.
• This download comes as a ZIP file selenium-X.XX.X.zip. Extract the contents of this ZIP file, including all the JAR files you will need to import into Eclipse later in this tutorial.
Configuring Eclipse IDE with Selenium WebDriver
1. Launch the eclipse.exe file inside the eclipse folder that you installed in the previous section.
2. Select the default location for the workspace.
3. Create a new project through File > New > Java Project. Give your project a name.
4. Right-click on the newly created project and then select New > Package, and give that package a name.
5. Create a new Java class under the package by right-clicking on it and then selecting New > Class. Your Eclipse IDE should look like the image below.
6. Right-click on the project and select Properties.
7. Click on Java Build Path.
8. Click on the Libraries tab, and then click Add External JARs…
9. Navigate to the location where you saved the extracted contents of “selenium-X.XX.X.zip” from the previous step.
10. Add all the JAR files inside and outside the libs folder. The Properties dialog should now look similar to the image below.
11. Click OK. Now you’re done importing Selenium libraries into your project.
Configuring Selenium WebDriver
To start executing the web application, we need to configure Selenium WebDriver with our code.
1. Download the Chrome browser driver from here.
2. Download the Chrome driver based on your system configuration.
3. Unzip the folder and keep it in a location (preferably in the project folder).
Create Your First Selenium Script
Let’s start with some fun stuff! Below are the final steps to complete this Selenium WebDriver tutorial.
1. First, we need to import the packages we’ll need as shown below. These will help us drive our scripts.
package basePackage
This is the package created before creating the Java class.
import org.openqa.selenium.By;
This package contains the By class which consists of locators by which the elements will be found from the web page.
import org.openqa.selenium.WebDriver;
This package contains the WebDriver class needed to instantiate a new browser.
import org.openqa.selenium.chrome.ChromeDriver;
This package contains the ChromeDriver class needed to instantiate a Chrome-specific driver onto the browser instantiated by the WebDriver class.
import org.openqa.selenium.support.ui.Select;
This package contains the Select class by which drop-downs can be handled.
2. In the class created, we now create a main function. This will be the entry point of the automated test execution.
public class BaseClass { public static void main(String args[]) throws InterruptedException {
3. Set the web driver location to where the driver is stored, from the Configuring the WebDriver section.
This defines that a property is set for a WebDriver, which enables the scripts to understand where to select a driver to start the execution.
System.setProperty("webdriver.chrome.driver", "C:\\Users\\utsav\\Downloads\\chromedriver_win32\\chromedriver.exe");
4. Create a driver object. This will instantiate the WebDriver object.
WebDriver driver = new ChromeDriver();
5. Initiate browser with the application URL
driver.get("/");
Sometimes the browser does not open in a maximized mode so you can use this to maximize browser use:
driver.manage().window().maximize();
6. Now let’s get the browser title and print the output:
String pageTitle = driver.getTitle(); System.out.println("Page title is: "+pageTitle);
7. You can perform click actions with the click() method. This will click on the Contact button on the page. The element is identified by relative XPath:
driver.findElement(By.xpath("//*[@id=\"main-header\"]/div[1]/div[3]/a")).click();
8. To enter text in the text field, you use the sendKeys() method. This will input text in a text box on the page. The element is identified by relative XPath:
driver.findElement(By.xpath("//input[@type='text' and @name='firstname']")).sendKeys("Utsav Jain");
9. You can also select a value from the drop-down using one these methods:
• By visible text
• By value
• By index
Select drpCountry = new Select(driver.findElement(By.name("role"))); drpCountry.selectByVisibleText("Executive Leadership"); drpCountry.selectByValue("Developer"); drpCountry.selectByIndex(2);
After tests are executed, you use the close() method to close the browser session:
driver.close();
Let’s Look at the Complete Code
package basePackage import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.Select; public class BaseClass { public static void main(String args[]) throws InterruptedException { System.setProperty("webdriver.chrome.driver", "C:\\Users\\utsav\\Downloads\\chromedriver_win32\\chromedriver.exe"); WebDriver driver = new ChromeDriver(); driver.get("/"); driver.manage().window().maximize(); String pageTitle = driver.getTitle(); System.out.println("Page title is: "+pageTitle); Thread.sleep(3000); driver.findElement(By.xpath("//*[@id=\"main-header\"]/div[1]/div[3]/a")).click(); Thread.sleep(3000); driver.findElement(By.xpath("//input[@type='text' and @name='firstname']")).sendKeys("Utsav Jain"); Select drpCountry = new Select(driver.findElement(By.name("role"))); drpCountry.selectByVisibleText("Executive Leadership"); drpCountry.selectByValue("Developer"); drpCountry.selectByIndex(2); driver.close(); } }
What Will You Do Next with Selenium WebDriver?
In this Selenium WebDriver tutorial, you learned how to install and configure the tool, as well as how to do some very basic UI testing automation with it. Selenium can also be used in automated visual testing.
If you’re new to testing automation and want to learn the basics, you’ll find Selenium WebDriver an excellent tool. I encourage you to explore how it can help you save time and improve your testing process.