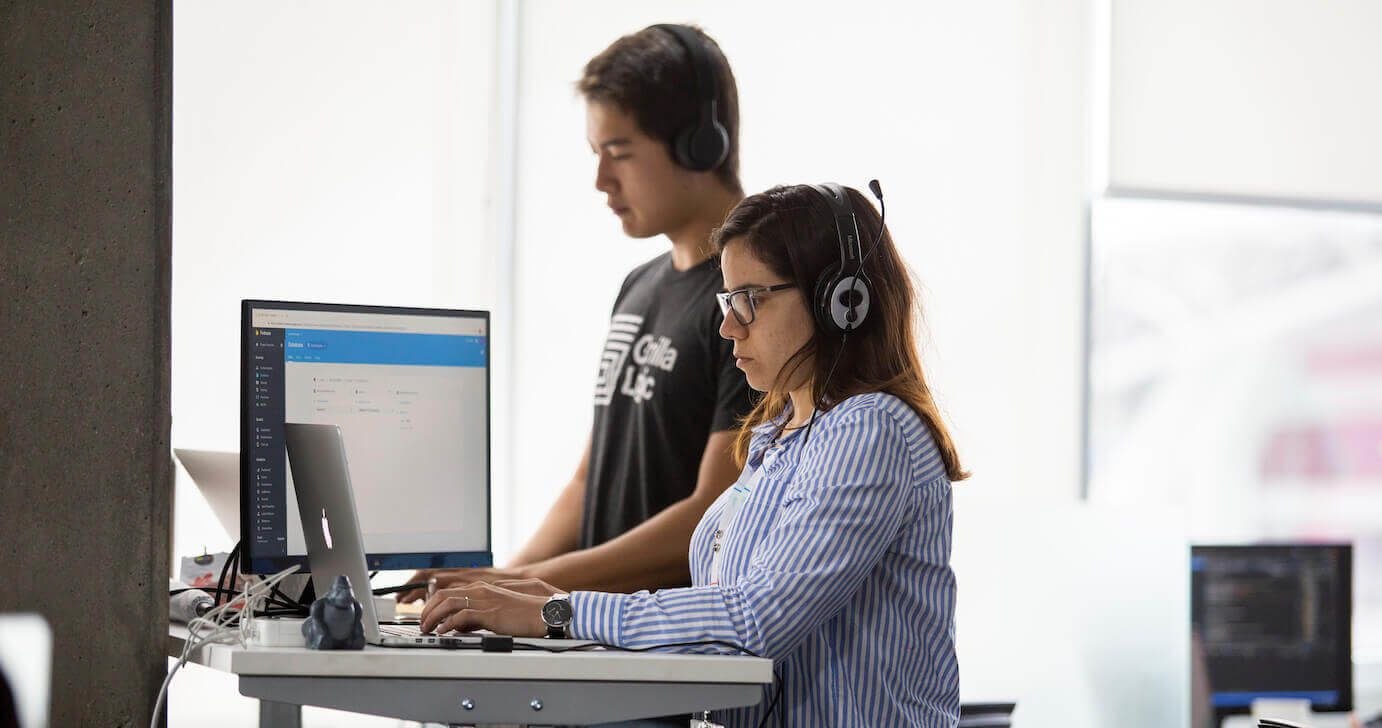
A Bitbucket Tutorial: Creating a Private Repository for an Android Library
When you work on a project with a development team, you need easy, secure ways to share code and resources. In this blog, I’ve created a Bitbucket tutorial to show you how to create a secure, shared library for all your project code and resources. With Bitbucket, a repository hosting service from Atlassian, you’ll also get tools for code management, project planning, and testing and deploying your projects. In addition, you can:
• Wrap up a third-party functionality.
• Expose any application layer or any other core component between your applications.
• Share all those resources between multiple projects.
For our Bitbucket tutorial, we’ll create and share a private Android library.
1. Configure Bitbucket
1. In Bitbucket, create a repo called “android_repo_test” as shown below.
2. Set up an SSH key, so that we can upload the module library to the repository later in this tutorial. You can find detailed documentation here. And here’s what you’ll do if on macOS:
a) Open terminal.
b) Type ssh-keygen
c) Press the Enter.
d) Enter and re-enter a passphrase when prompted.
If you don’t want to type your password each time you use the key, you’ll need to add it to the ssh-agent like this:
a) Type eval `ssh-agent`
b) Press the Enter.
c) Type ssh-add -K ~/.ssh/<private_key_file> ( where private key file could be id_rsa depending on the name of the file you created before after typing ssh-keygen )
Note: (macOS only) So that your computer remembers your password each time it restarts, open (or create) the ~/.ssh/config file and add these lines to the file:
Host *
UseKeychain yes
3. Once you’ve set the local SSH key, you must add it to Bitbucket. Follow the Bitbucket documentation to Add the public key to your Bitbucket settings in this SSH Key set up.
2. Android library (AAR)
Let’s create the Android library for our Bitbucket tutorial.
1. Create a new .gradle file named uploadLibrary.gradle in the same folder of your module build.gradle
2. Push our project to Bitbucket:
a) Open terminal.
b) cd /path/to/your/repo
c) git init
d) git add –all
e) git commit -m “Initial Commit”
f) git remote add origin https://<your_bitbucket_user>@bitbucket.org/<your_bitbucket_user /android_repo_test.git
g) git push -u origin master
3. Add the following code in the gradle.properties (use your own credentials for COMPANY).
major = 1 minor = 0 patch = 0 ARTIFACT_NAME=myLibrary ARTIFACT_PACKAGE=com.library.mylibrary ARTIFACT_PACKAGING=aar COMPANY=myBitbucket REPOSITORY_NAME=android_repo_test
4. Add this next code in the module build.gradle after any apply plugin:
You have and before the android {} block (you should not add this in the build.gradle from the root Project; in this example, that would be the build.gradle from Project: MyLibrary).
apply from: 'uploadLibrary.gradle'
3. Wagon-git
To upload the artifacts to the Git repository, we’ll use a Maven plugin called Wagon-Git.
1. In the uploadLibrary.gradle, add the following code:
apply plugin: 'maven' repositories { maven { url "https://raw.github.com/synergian/wagon-git/releases"
}
} configurations { deployLibrary
} dependencies { deployLibrary "ar.com.synergian:wagon-git:0.2.5"
} uploadArchives { repositories.mavenDeployer { configuration = configurations.deployLibrary repository(url: 'git:master: //git@bitbucket.org:' + COMPANY + '/' + REPOSITORY_NAME + '.git') pom.project { groupId = ARTIFACT_PACKAGE version = "${major}.${minor}.${patch}" artifactId = ARTIFACT_NAME packaging ARTIFACT_PACKAGING } } }
Feel free to commit the rest of the files to your repository.
2. Open the terminal and inside your project run this command:
./gradlew uploadArchives
3. You will see the commit in your repository:
4. Troubleshooting
I’ve found that most errors are related to the SSH key configuration, incorrect credentials, or Git conflicts.
If you have issues running the ./gradlew uploadArchives command, try running the command to check for log errors:
./gradlew –info uploadArchives
Check the configuration of your SSH key by running this command:
ssh -T git@bitbucket.org
If “wagon-git” isn’t able to push the files to the repository (a known issue that will sometimes come up):
1. Delete then add the “wagon-git” duplicate folder(s) in the Temp folder.
a) In a macOS, you’ll need to go to the /private/var/folders. For reference go here.
b) For quick access to your Temp folder type in your Terminal: Open $TMPDIR
5. Consuming the Library
Bitbucket App Password
In order to consume the library, we must set up an App Password so we don’t have to use our personal Bitbucket password. Below, I’ve included a quick summary of the steps. Check the Bitbucket documentation for more detailed information.
1. From your profile Bitbucket settings, follow these screens:
2. Add Read permission for our app to access the repository.
3. Then copy-paste the app password in a secure place as we will need it later on in this tutorial.
Android Application
1. Create a new Android application or use one you already have.
2. Add the following code in the gradle.properties (Use here your own credentials for COMPANY)
COMPANY=myBitbucket REPOSITORY_NAME=android_repo_test
3. In your local.properties files add this code, where username is your Bitbucket username and password is your App Password:
USERNAME=yourusername PASSWORD=apppassword
4. Now, in your main root build.gradle project add this code:
Properties properties = new Properties() properties.load(project.rootProject.file('local.properties').newDataInputStream()) def USERNAME = properties.getProperty('USERNAME') def PASSWORD = properties.getProperty('PASSWORD') allprojects { repositories { google() jcenter() maven { credentials { username USERNAME password PASSWORD } authentication { basic(BasicAuthentication) } url "https://api.bitbucket.org/2.0/repositories/" + COMPANY + "/" + REPOSITORY_NAME + "/src/master" } } }
5. Add this code inside the build.gradle dependencies block:
implementation ('com.library.mylibrary:mylibrary:1.0.0')
Note: Do not commit your local.properties file!!
That’s it!!! You can now create libraries and share them with your team.
Now It’s Time to Build on What You’ve Learned
In our Bitbucket tutorial, you might have noticed the only branch we used was “master” and we didn’t use versioning. Instead, we focused just on the most basic steps, including how to:
• Create a private library.
• Include that library in your project.
• Avoid boilerplate code using a unique library.
There’s so much more you can do, and I encourage you to experiment. Try adding versioning for the library. Select another branch. Make a gradle script to simplify some of the code. Explore all of Bitbucket’s features so you can discover the best way to use it with your team.
To understand more about the different types of dependencies you can use in your project and their differences take a look here.
And, a final word. In every development project, testing is fundamental. When you’re working with a team, and creating and sharing libraries, your team needs to work with your library without having issues, spending time looking for errors, or trying to debug your code. Build your library with testing best practices so your team gets the most value from working with it.
What has helped you create a shared private library? Please try this tutorial, share your private library with your team, and then share what you’ve learned in the comments below.